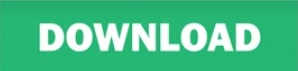
To learn more about Python dictionaries, check out the official documentation here.
Python sort list of dictionaries on key how to#
You learned how to do this using the ems() method, a Python for loop, a list comprehension, and a lambda function. In this tutorial, you learned how to sort a Python dictionary by its values. More of a visual learner, check out my YouTube tutorial here. Want to learn more about Python list comprehensions? Check out this in-depth tutorial that covers off everything you need to know, with hands-on examples. Let’s see what this looks like in practice: # Sorting a Dictionary by its values using sorted() If you put things in a list in a certain order, they stay in that order. In essence, it’s a function that’s evaluated in order to compare against how to sort.īecause we want to compare against the items’ values, we will use key=item.get, which returns a key’s value.

The key is the method by which to sort data.

Now it’s certainly possible that right now you do in fact have a good use case for sorting a dictionary (for example maybe you’re sorting keys in a dictionary of attributes), but keep in mind that you’ll need to sort a dictionary very rarely.
Python sort list of dictionaries on key code#
If you can get away with using a list of tuples in your code (because you don’t actually need a key-value lookup), you probably should use a list of tuples instead of a dictionary.īut if key lookups are what you need, it’s unlikely that you also need to loop over your dictionary. They’re very fast at retrieving values for keys.īut dictionaries take up more space than a list of tuples.

In fact, when you’re considering looping over a dictionary you might ask “do I really need a dictionary here”?ĭictionaries are used for key-value lookups: you can quickly get a value given a key. When you’re about to sort a dictionary, first ask yourself “do I need to do this”? create a function named getdata, that takes two arguments, Data and Key. We can’t sort a dictionary in-place, but we could get the items from our dictionary, sort those items using the same technique we used before, and then turn those items into a new dictionary: Unlike lists, there’s no sort method on dictionaries.
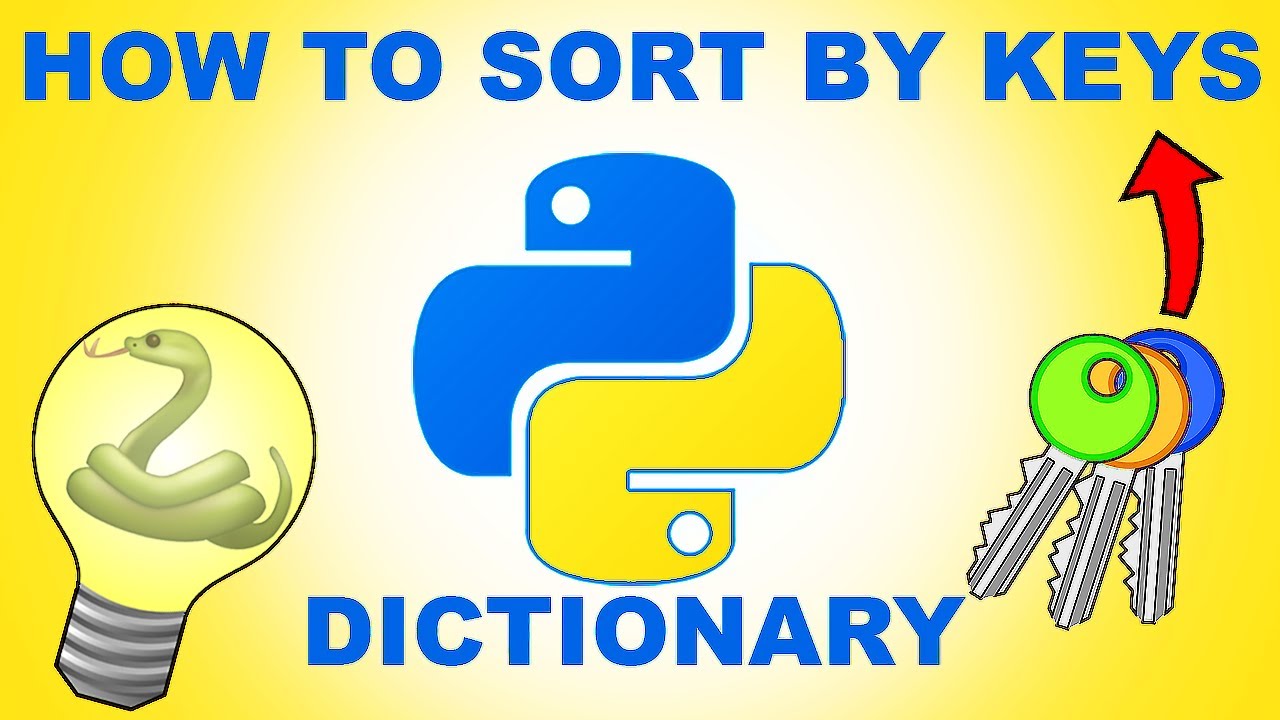
What if we already have our items in a dictionary and we’d like to sort that dictionary? So as long as the keys are comparable to each other with the less than operator ( <), sorting 2-item tuples of key-value pairs should always sort by the keys. The keys in a dictionary should always compare as unequal (if two keys are equal, they’re seen as the same key). You might be thinking: it seems like this sorts not just by keys but by keys and values. I’ve written an article on tuple ordering that explains this in more detail. Def compare_two_item_tuples ( a, b ): """This is the same as a < b for two 2-item tuples.""" if a != b : # If the first item of each tuple is unequal return a < b # Compare the first item from each tuple else : return a < b # Compare the second item from each tuple Note that from Python 3.7 and above, dictionaries are ordered by their keys by default.
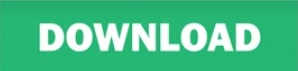